50. Remote debugging¶
Running AGX Dynamics embedded into a simulation framework, it can be sometimes hard to understand what is going on during a simulation. Therefore there is a feature which allows for some remote viewing capabilities.
In short, it is utilizing the serialization of a agxSDK::Simulation
over a network port. This means that the whole simulation will be serialized, transmitted over the network and de-serialized and visualized in the agxViewer application.
Note
The Remote debugging feature should not be part of any production code, but rather as a way of looking into a running simulation. Enabling remote debugging will greatly affect the performance.
First, let´s have a look at how this works by using the command arguments for agxViewer.
50.1. Debugging agxViewer¶
Start a command prompt with the correct AGX runtime environment setup.
Next, start another command window (you will use one for the remote viewer and one for running the simulation).
From the first command prompt:
> agxviewer --server --serverTimeStep 0.1 --serverWait 0 data/python/overheadConveyor.agxPy
[Warning: agxSDK::Simulation::setEnableRemoteDebugging] Starting debugging server at port: 9999
Loading scene took 0.705391 sec
and from the second command prompt write:
> agxviewer --connect localhost -p --renderDebug 1
Connecting to remote server at localhost port: 9999
Trying to connect to server...
Connected.
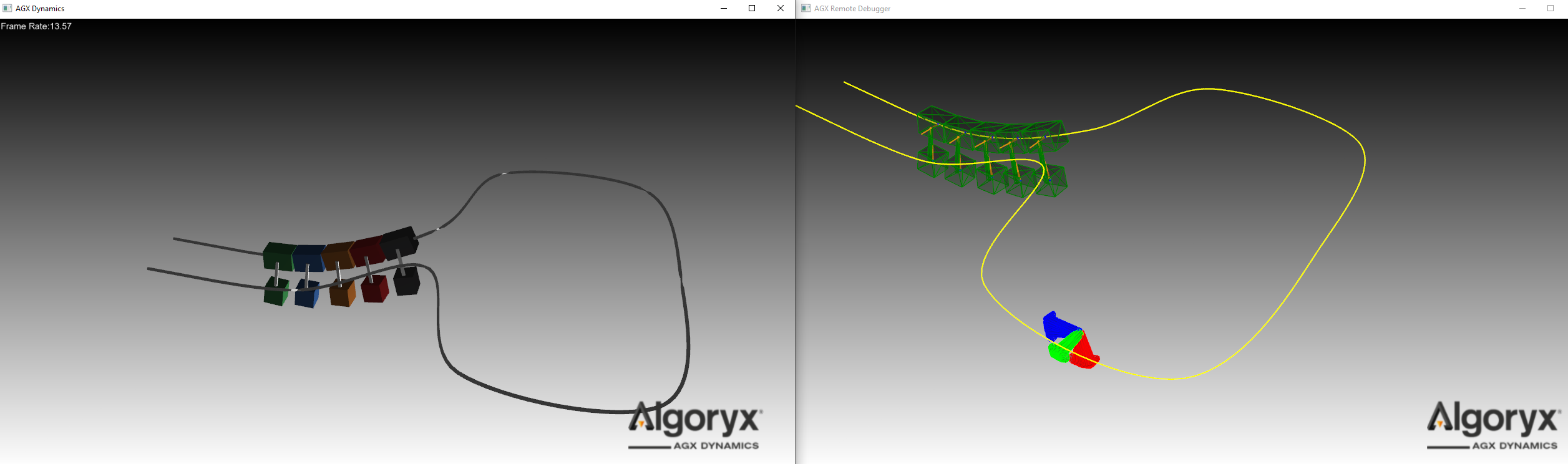
Fig. 50.1 The image to the left show the running simulation and the one to the right show the remote debugger view.¶
Lets look at the arguments of the two commands. On the server side we have:
--server
- Indicates that a port should be setup where the server will wait for a connection. The port can be changed using the--serverPort <portnum>
argument. Default is 9999--serverTimeStep 0.1
- specifies the rate by which the simulation will be streamed over the network connection in ms. This should be a multiple of the current simulation time step.--serverWait 0
- If this is set to 0 (disabled) the server will not wait for a ready signal from the client and instead just push data onto the network. Hence synchronization between client and server will not be upheld.and finally the script that should be executed.
On the client side we have the following arguments:
--connect localhost
- This specifies the network ip adress to where the server is running. In this case localhost (127.0.0.1)-p
or--startPaused
- This is important as it will start agxViewer in paused mode. This will show the data being streamed into the client. If this was omitted, or if you press ‘e’ key to start the simulation, you would no longer stream data into the client, instead you are running the actual simulation locally. This is enabled by the fact that we are doing full de-serialization of the simulation.--renderDebug 1
- This will enable the debug renderer so that we can actually see the parts of the simulation.
50.2. Enabling remote debugging in an application¶
The remote debugging feature can be enabled for any agxSDK::Simulation
instance.
agxSDK::SimulationRef simulation = new agxSDK::Simulation();
// Specify the rate by which we will send data over to the server. Should be a multiple of the simulation time step
double serverTimeStep = simulation->getTimeStep() / 2.0;
// Specify the TCP port on which the server will listen to the connection
agx::Int16 serverPort = 12345;
// Should we compress (zip) the data before sending?
bool compressData = false;
// Lets not wait for client to be ready for new data. Just send new packages as fast as we can
bool waitForClient = false;
simulation->setRemoteDebuggingTimeStep(serverTimeStep);
// Enable the remote debugging
simulation->setEnableRemoteDebugging(true, serverPort, compressData, waitForClient);
50.3. Performance of remote debugging¶
Due to the full serialization of the simulation state each time it is being synchronized, the performance can be very much affected. Large objects such as heightfield, triangle meshes and terrains can lead to very slow remote debugging.
In those cases where you cannot achieve acceptable performance you can disable serialization for some of the larger objects.
For example if you have a heightfield as a seabed, but you are mostly interested in looking at some mechanical simulation above the heightfield, then you can call the Serializable::setEnableSerialization(bool)
method.
// Disable serialization for a agxTerrain::Terrain
terrain->setEnableSerialization(false);
// This will lead to better performance in remote debugging.
// Note that you probably have to loop through your geometries and look for
// triangle meshes/heightfields that you want to disable for serialization.
heightfield_geometry->setEnableSerialization( false );