17. AGX Cable damage¶
An important factor in cable application design is cable wear. The cable damage component of the agxCable module provides a way to estimate damage caused to the cable during the simulation. Effects such as tension, deformation, and contacts are taken into account by the damage estimation model and applied to each cable segment individually.
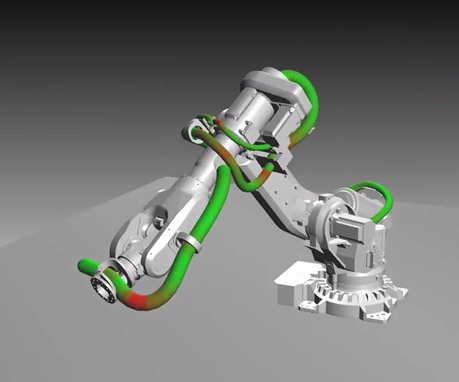
Fig. 17.1 Robot with several cables. Current cable damage visualized by color.¶
17.1. Basic usage¶
The damage estimation is performed by creating an instance of the agxCable::CableDamage
class. It uses the simulation result of a cable to estimate damage and wear inflicted on the cable. The following code should be executed before the simulation starts to gather damage estimation for a cable named cable.
agxCable::CableDamageRef damage = new agxCable::CableDamage();
cable->addComponent(damage);
During or after the simulation the estimated damages can be accessed via instances of the SegmentDamage class, which the* CableDamage* instance provides. Damage is estimated per cable segment, and there is one SegmentDamage instance per segment of the simulated cable. We can read the damage accumulated over the simulation so far for a particular cable segment with the following code.
agx::Real wear = cableDamage->getAccumulatedDamageAt(segmentIndex).total();
The damage inflicted to a particular cable segment during the most recent simulation step is accessed using the following code.
agx::Real newWear = cableDamage->getCurrentDamageAt(segmentIndex).total();
In addition to the total damage, it is possible to read the contribution from the individual damage estimation sources for a particular segment. To better understand the available sources we must first understand the damage estimation model.
17.2. Damage estimation model¶
Cable damage is estimated each time step from a number of contribution sources. Each source is scaled according to a weight associated with the source and the weighted values are summed to form a total damage contribution for that time step.
The sources are categorized into four groups: deformation, deformation rate, tension, and contact force. The first three groups are further categorized based on the direction of deformation (or tension): bend, twist, and stretch. The fourth group, contact force, is split in normal force and friction force. This results in a total of eleven (3x3+1x2) damage estimation sources.
Deformation, deformation rate and tension have a threshold each, below which a deformation/tension gives no contribution to the damage estimation.
The complete damage estimation formula is as follows:
W |
Weighting factor |
T |
Tension |
R |
Deformation rate |
F |
Contact force |
b |
Along bend direction |
t |
Along twist direction |
s |
Along stretch direction |
n |
Along contact normal |
f |
Along contact tangent |
0 |
Deformation threshold |
The following image shows the contribution of the different sources:
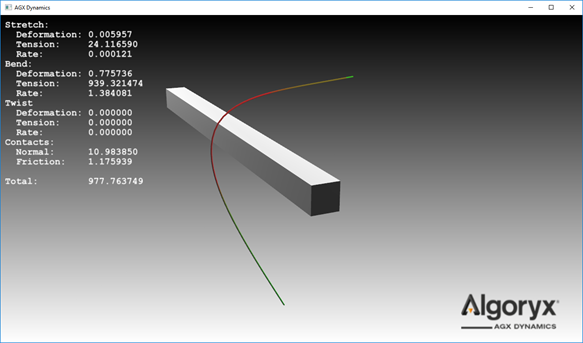
Damage estimation is tracked per cable segment. Dynamic cable properties that are defined as a relationship between two consecutive cable segments, such as tension and deformation, are distributed evenly over the two segments and thus the segment’s damage contribution becomes the average of the damage contributions at the segment’s two end points.
17.3. Parameters¶
The cable damage model contains two types of parameters: weights and thresholds. The weights control how much each individual damage estimation source should contribute to the final total. The thresholds control the smallest deformation required for the deformation related damage estimation sources to take effect. The following code shows an example of how to set some of these parameters. The damage estimation model section details the available weights and thresholds.
damage->setStretchDeformationWeight(100.0);
damage->setTwistDeformationWeight(30.0));
damage->setBendRateWeight(10);
damage->setTwistTensionWeight(0.04);
damage->setFrictionForceWeight(2.0);
damage->setStretchThreshold(0.0);
damage->setBendThreshold(0.1);
The patterns are set[Bend|Twist|Stretch][Deformation|Rate|Tension]Weight, set[Normal|Friction]ForceWeight, and set[Bend|Twist|Stretch]Threshold.
Changing the parameters will affect simulation steps taken after the change, but not damage estimates for time steps already completed. This makes it possible to alter the cable damage parameters over time to account for changes to the cable or the environment during the simulation.
The cable damage calculations and its parameters do not interact in any way with the cable material parameters and accumulated cable damage does not influence the simulation behavior of the cable.
17.4. Tutorial¶
The C++ tutorial tutorial_cable_damage.cpp shows more details on how to set up a scene with cable damage.