21. AGX Model: Tire¶
agxModel::TwoBodyTireModel is an implementation of a tire model with two separate rigid bodies; the tire and the rim. The user has control over the deformation of the different degrees of freedom by setting stiffness and damping:
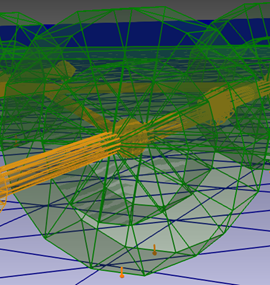
Creating a TwoBodyTireModel requires two bodies, one for the rim and one for the rigid body. It is assumed that the two bodies are positioned and added to the simulation before or after the creation of the TwoBodyTireModel
agx::Real tireRadius = 1; // Not important, but can give information of
agx::Real rimRadius = 0.5; // amount of deformation of tire.
agx::Real stiffness = 2E6;
agxModel::TwoBodyTireModelRef tire =
new agxModel::TwoBodyTireModel(tireBody, tireRadius, rimBody, rimRadius );
21.1. Stiffness¶
The stiffness of the tire will control the amount of deformation for the tire. There are four degrees of freedom for which the stiffness can be specified.
The unit for translational stiffness is force/displacement (if using SI: N/m). The unit for rotational stiffness is torque/angular displacement (if using SI: Nm/rad)
// Configure the deformation stiffness for all DOF of the tire
tire->setStiffness(stiffness*2, agxModel::TwoBodyTire::RADIAL)
tire->setStiffness(stiffness*10, agxModel::TwoBodyTire::LATERAL)
tire->setStiffness(stiffness*4, agxModel::TwoBodyTire::BENDING)
tire->setStiffness(stiffness*0.1, agxModel::TwoBodyTire::TORSIONAL)
21.2. Damping¶
The damping corresponds to the rate of which the deformation should be restored. It has the same degrees of freedom as the stiffness.
The unit for translational damping is force * time/displacement (if using SI: Ns/m). The unit for the rotational damping coefficient is torque * time/angular displacement (if using SI: Nms/rad)
dampingCoefficient = 70000
tire.setDamping(dampingCoefficient*2, agxModel.TwoBodyTire.RADIAL)
tire.setDamping(stiffness*10, agxModel.TwoBodyTire.LATERAL)
tire.setDamping(stiffness*4, agxModel.TwoBodyTire.BENDING)
tire.setDamping(stiffness*0.1, agxModel.TwoBodyTire.TORSIONAL)